Perl arrays let you use tabular data or iterate over a list of values, for example, a set of data points for a question. In addition, the Question Editor supports a special array called @dat.
Perl array variables are prefixed by the at sign (@). In addition, all Perl variable names must follow these rules:
- Variable names must contain only letters (a-z, A-Z), underscores (_), and numeric digits (0-9).
- The first character of a variable name must be a letter (a-z, A-Z) or underscore (_).
- Variable names are case-sensitive, so myvariable is not the same as MyVariable.
Some variable names are used by WebAssign. These variables are listed in the documentation.
One-Dimensional Arrays
One-dimensional arrays are like grocery lists. For example:
wine
cheese
bread
In Perl, you enclose the list in parentheses and separate individual list items with commas. For example:
@groceries = ('wine', 'cheese', 'bread');
To refer to a single element in the array, specify the array variable as a scalar with an index indicating which element you are referring to. The index starts with 0 and follows the variable name in brackets. For example:
The second item on my grocery list is <eqn $groceries[1]>.
You can mix numeric and text data in an array.
@one_to_ten = (1..10);
Two-Dimensional Arrays
Two-dimensional arrays let you work with tabular data. For example:
Mercury | 0.39 |
Venus | 0.72 |
Earth | 1 |
Mars | 1.52 |
Jupiter | 5.20 |
Saturn | 9.54 |
Uranus | 19.18 |
Neptune | 30.06 |
In Perl, you enclose the entire table in parentheses, enclose each row in brackets, and separate both rows and table cells with commas. For example:
@dat = ( ['Mercury' , 0.39 ],
['Venus' , 0.72 ],
['Earth' , 1 ],
['Mars' , 1.52 ],
['Jupiter' , 5.20 ],
['Saturn' , 9.54 ],
['Uranus' , 19.18],
['Neptune' , 30.06] );
To refer to an element in a two-dimensional array, specify the array variable as a scalar with two indexes indicating the row and column of the element you are referring to. The index values start with 0 and follow the variable name in brackets. For example:
The average distance from the Sun to <eqn $dat[4][0]> is <eqn $dat[4][1]> AU.
The @dat Array
WebAssign provides support for viewing one- and two-dimensional array values in the Question Previewer when you use an array named @dat. If your question contains the @dat array, the Question Previewer displays an additional tab named Array, which displays the contents of the array in addition to the question code and a preview of your question.
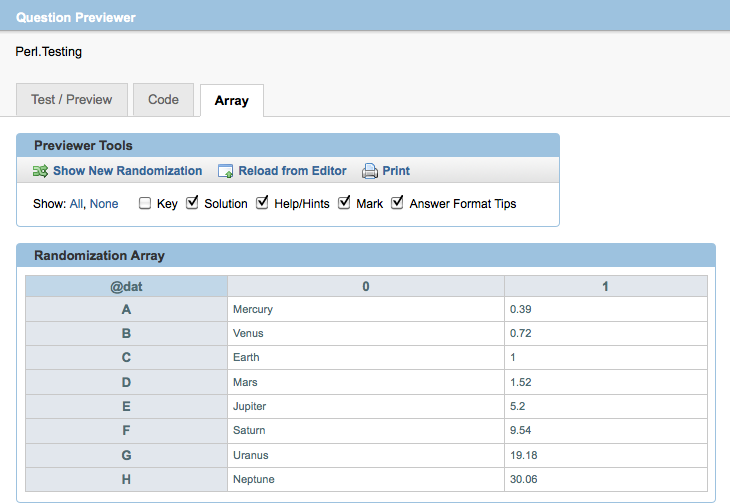
For example, in the array shown above, $dat[2][0] is "Earth" but $dat[C][0] would be parsed as $dat[0][0] and return "Mercury."
In all other respects, the @dat array is a normal array and can contain whatever values you want.
Example Question Using Array to Define Possible Answers
The following table summarizes an actual question.
QID | |
---|---|
Name | |
Mode | Fill-in-the-Blank |
Question |
|
Answer |
|
Display to Students |
![]() |
Example Question Using Array and Randomization
The following table summarizes an actual question.
QID |
|
---|---|
Name |
|
Mode | Multiple-Choice |
Question |
|
Answer |
|
Display to Students |
![]() |